Serialization is the process of converting complex data structures into formats that can be easily transmitted and stored. Such as JSON-LD, which is the default file format, or XML, CSV and other universally accessible ones. The reverse process, in which the data structure is recreated from a serialized state is known as deserialization.
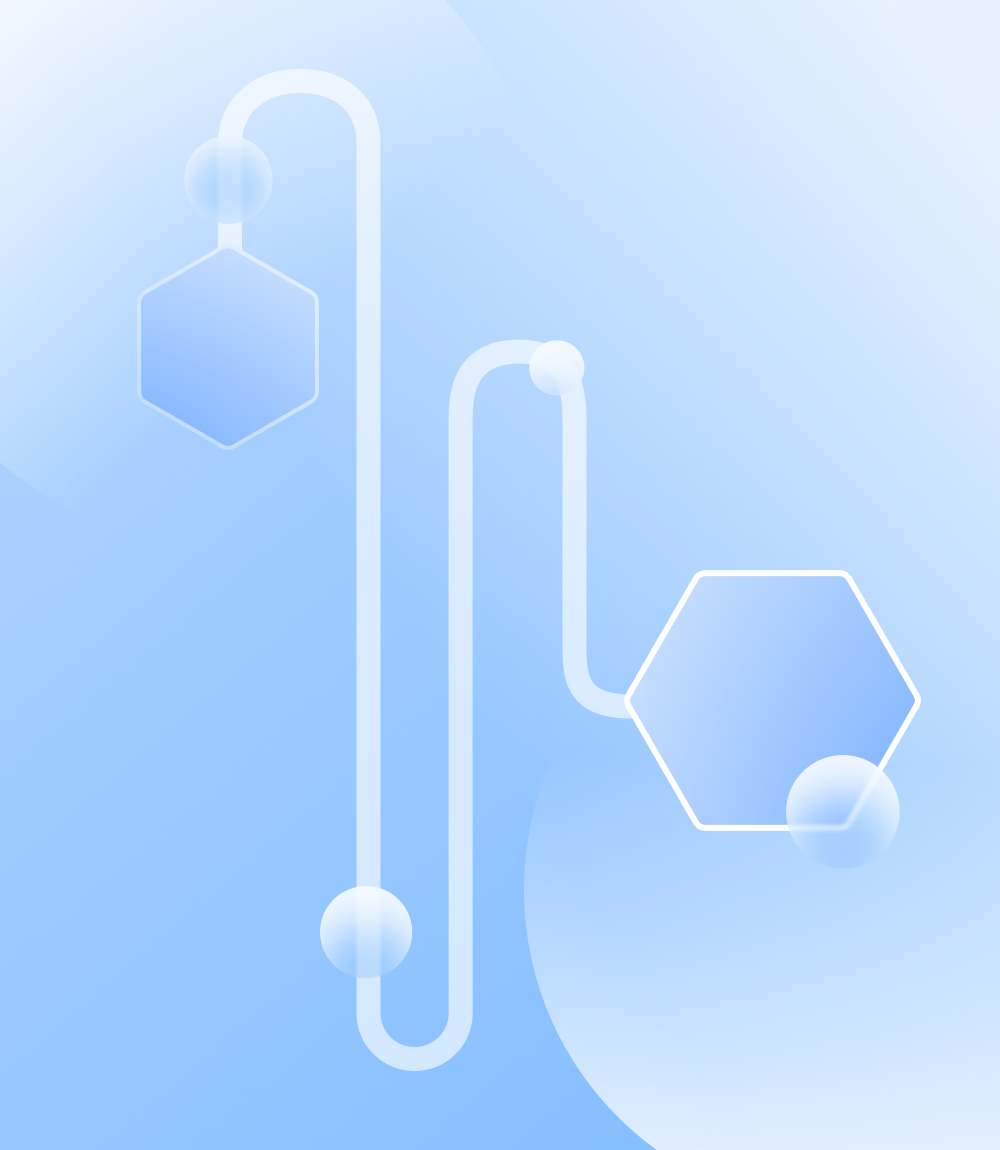
Both of these processes are essential in facilitating communication between different services.
So, how does this translate to using API Platform?
Serialization in API Platform
Consists of two stages; normalization and encoding.
Normalization transforms an object into a PHP array, which is then encoded and becomes a JSON string.
Deserialization in API Platform
Consists of two stages; decoding and denormalization.
Here, the JSON string is first decoded to become a PHP array and then transformed into an object through denormalization.
Serialization groups in API Platform
Serialization groups in API Platform allow you to choose which fields of an object should be serialized or deserialized. By default, they are defined by using attributes (PHP 8.) or “docstrings” (PHP 7.). The GET method is used to collect data from API, while the PUT, PATCH, and POST methods are used to modify or create new resources.
Examples of using serialization groups can be found below:
<?php
declare(strict_types=1);
namespace App\Entity;
use ApiPlatform\Core\Annotation\ApiResource;
use Symfony\Component\Serializer\Annotation\Groups;
#[ApiResource(
normalizationContext: ['groups' => ['ticket.read']],
denormalizationContext: ['groups' => ['ticket.write']],
)]
class Ticket
{
public int $id;
#[Groups(['ticket.read', 'ticket.write'])]
public string $name;
#[Groups(['ticket.read'])]
public string $description;
...
}
As shown above, either “normalizationContext” or “denormalizationContext” should be added to each entity as it defines serialization groups. Next, in the “$name” field, you can specify the group. For example, when using “ticket.read”, API will retrieve this field through the GET method. To employ the POST/PUT/PATCH methods, use the “ticket.write” group.
Serialization can also be used to handle relations between objects in an API. By default, API platform retrieves relations in byte streams corresponding to the IRI standard which leads directly to a specific resource.
What’s more, serialization groups can be used for custom operations. Below, we present an example of this.
<?php declare(strict_types=1); namespace App\Entity; use ApiPlatform\Core\Annotation\ApiResource; use Symfony\Component\Serializer\Annotation\Groups; #[ApiResource(normalizationContext: ['groups' => ['ticket.read']])] #[Get] #[Put(normalizationContext: ['groups' => ['ticket.put']])] class Ticket { public int $id; #[Groups(['ticket.read', 'ticket.write'])] public string $name; #[Groups(['ticket.put'])] public string $description; ... }
How to decorate retrieved data in API platform?
Data retrieved by API Platform can also be decorated. This makes it possible to adjust some of the fields. Below, we present how to change the “name” field and add uniqid().
<?php
namespace App\Serializer;
use Symfony\Component\Serializer\Normalizer\DenormalizerInterface;
use Symfony\Component\Serializer\Normalizer\NormalizerInterface;
use Symfony\Component\Serializer\SerializerAwareInterface;
use Symfony\Component\Serializer\SerializerInterface;
final class TicketNormalizer implements NormalizerInterface, DenormalizerInterface, SerializerAwareInterface
{
private NormalizerInterface $decorated;
public function __construct(NormalizerInterface $decorated)
{
if (!$decorated instanceof DenormalizerInterface) {
throw new \InvalidArgumentException(sprintf('The decorated normalizer must implement the %s.', DenormalizerInterface::class));
}
$this->decorated = $decorated;
}
public function supportsNormalization($data, $format = null): bool
{
return $this->decorated->supportsNormalization($data, $format);
}
public function normalize($object, $format = null, array $context = []): array
{
$data = $this->decorated->normalize($object, $format, $context);
if (is_array($data)) {
$data['name'] = sprintf('%s - %s', $data['name'], uniqid());
}
return $data;
}
public function supportsDenormalization($data, $type, $format = null): bool
{
return $this->decorated->supportsDenormalization($data, $type, $format);
}
public function denormalize($data, string $type, string $format = null, array $context = [])
{
return $this->decorated->denormalize($data, $type, $format, $context);
}
public function setSerializer(SerializerInterface $serializer): void
{
if($this->decorated instanceof SerializerAwareInterface) {
$this->decorated->setSerializer($serializer);
}
}
}