When developing a rest API, it often happens that you want control over which users have the access to a specific resource. The usage of user roles can cover some cases. But what if you want to restrict the access not based on a given role, but some other dependencies? Sometimes the use cases you need to cover become quite complicated, other times they are simple, but repetitive. What allows you to control the access in a clean, un-repetitive way is called voters.
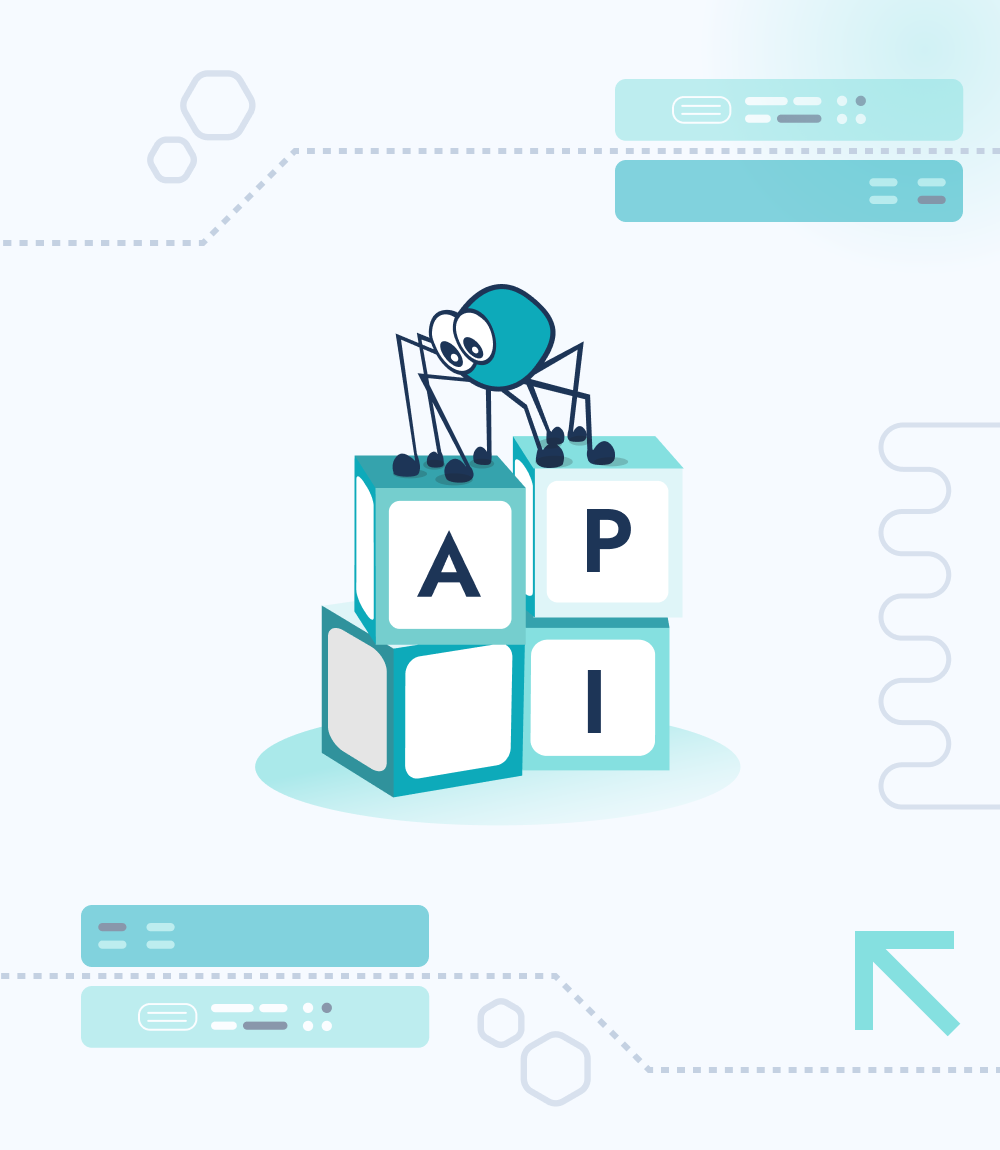
What Is a Voter?
Citing the Symfony docs, “Voters are Symfony's most powerful way of managing permissions. They allow you to centralize all permission logic, then reuse them in many places.”
What does it mean in practice? Voters are classes that extend the Symfony voter abstract class, and you can use them in order to enable access for the endpoint in the circumstances specified by you however you need it.
What Does a Voter Look Like?
class ShopVoter extends Voter
{
protected function supports(string $attribute, mixed $subject): bool
{
...
}
protected function voteOnAttribute(
string $attribute,
$subject,
TokenInterface $token
): bool {
...
}
}
In the provided example, we have created a voter that will be used to control access to the “Shop” resource.
The voter implementation needs to only implement two functions: supports and voteOnAttribute.
supports
This function needs to return true or false depending if we want to use this voter in the incoming request.
The most common usage of the voters is to create a Voter for each resource, and check whether the “$subject” is of the corresponding type, and if the attribute we are voting on is handled:
public const CREATE = 'create';
public const READ = 'read';
private const SUPPORTED_ATTRIBUTES = [
self::CREATE,
self::READ,
];
protected function supports(string $attribute, mixed $subject): bool
{
return
$subject instanceof Shop &&
in_array($attribute, self::SUPPORTED_ATTRIBUTES)
;
}
Of course this logic can be modified to match your needs.
voteOnAttribute
Function voteOnAttribute is responsible for finding out if we want to enable access to the given resource based on the attribute, the subject and a $token implementing Symfony’s TokenInterface that is given to us based on the configured authentication.
protected function voteOnAttribute(string $attribute, $subject, TokenInterface $token): bool
{
/** @var User|null|string $user */
$user = $token->getUser();
if (!$user instanceof User) {
return false;
}
switch ($attribute) {
case self::CREATE:
return $this->authorizationChecker->isGranted('ROLE_ADMIN');
case self::READ:
return $this->shopService->isUserAShopManager($user, $subject);
}
return false;
}
What’s really handy is that you can use dependency injection in order to use other services inside of your voter as shown above! In the default configuration, it’s enough to just add the required services to the voter’s “__construct” method.
How to Use the Custom Voter in API Platform?
The only thing you need to do is to add a “security” to your endpoint definition. You can use Is_granted by providing the “attribute” as the first argument, and the resource object as the second argument:
App\Entity\Shop:
itemOperations:
get:
security: is_granted('read', object)
post:
security: is_granted('create', object)